I already had the Dreamlands one in French, but I recently grabbed both in English (they had a decent price on eBay!) For a long time it was my only systemless RPG supplement and I loved how that, with its weird illustrations, set it apart!
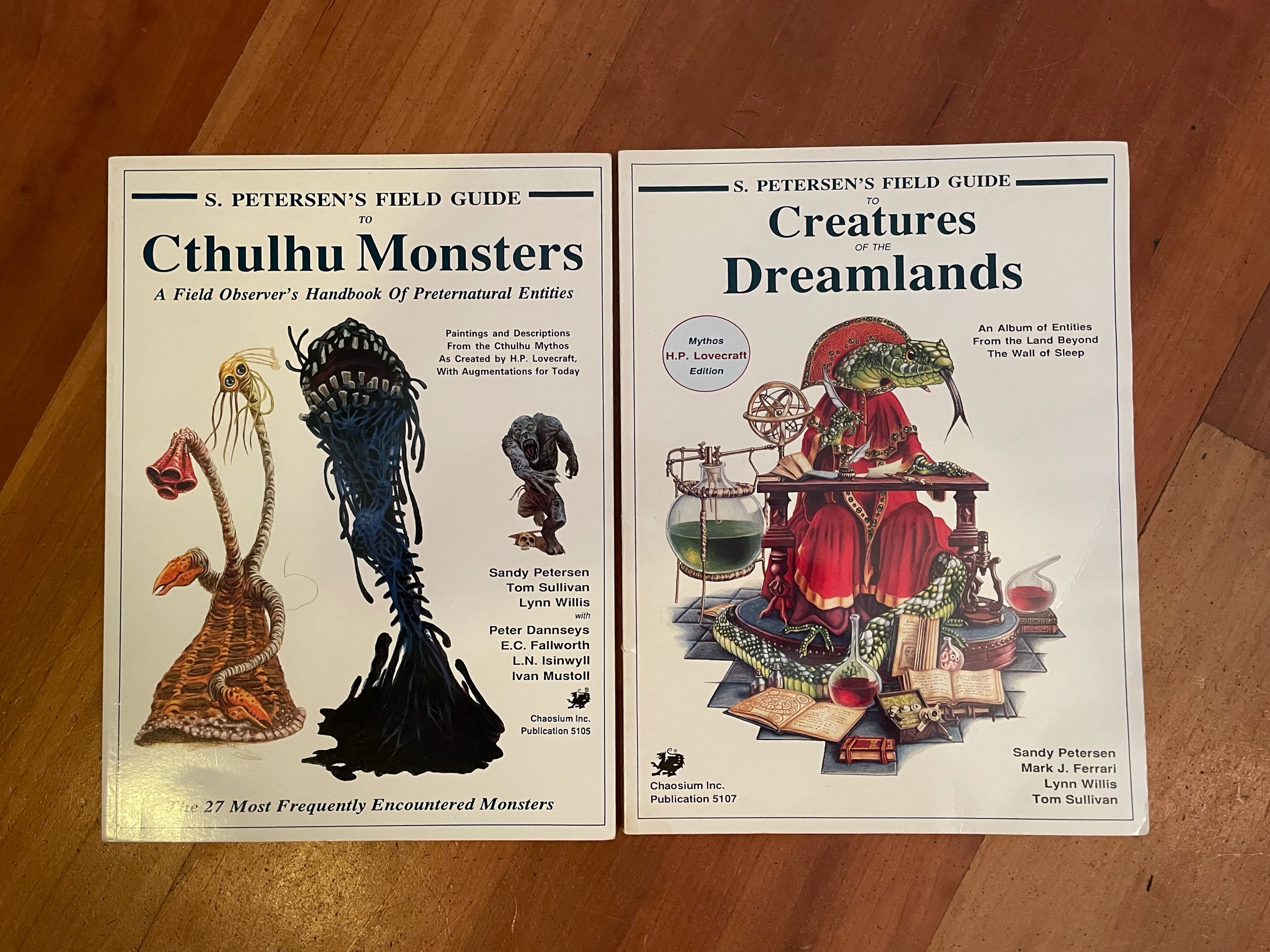
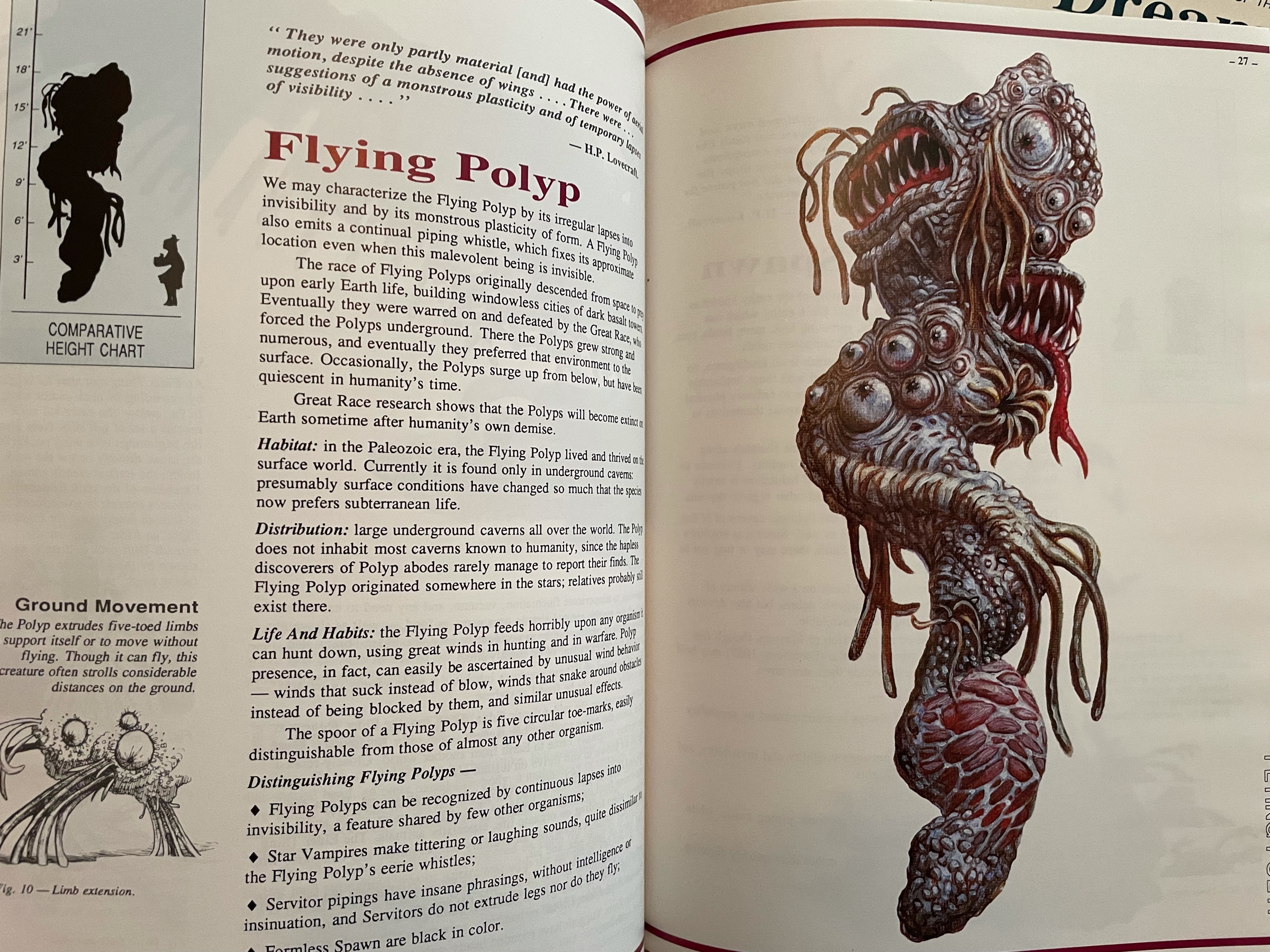
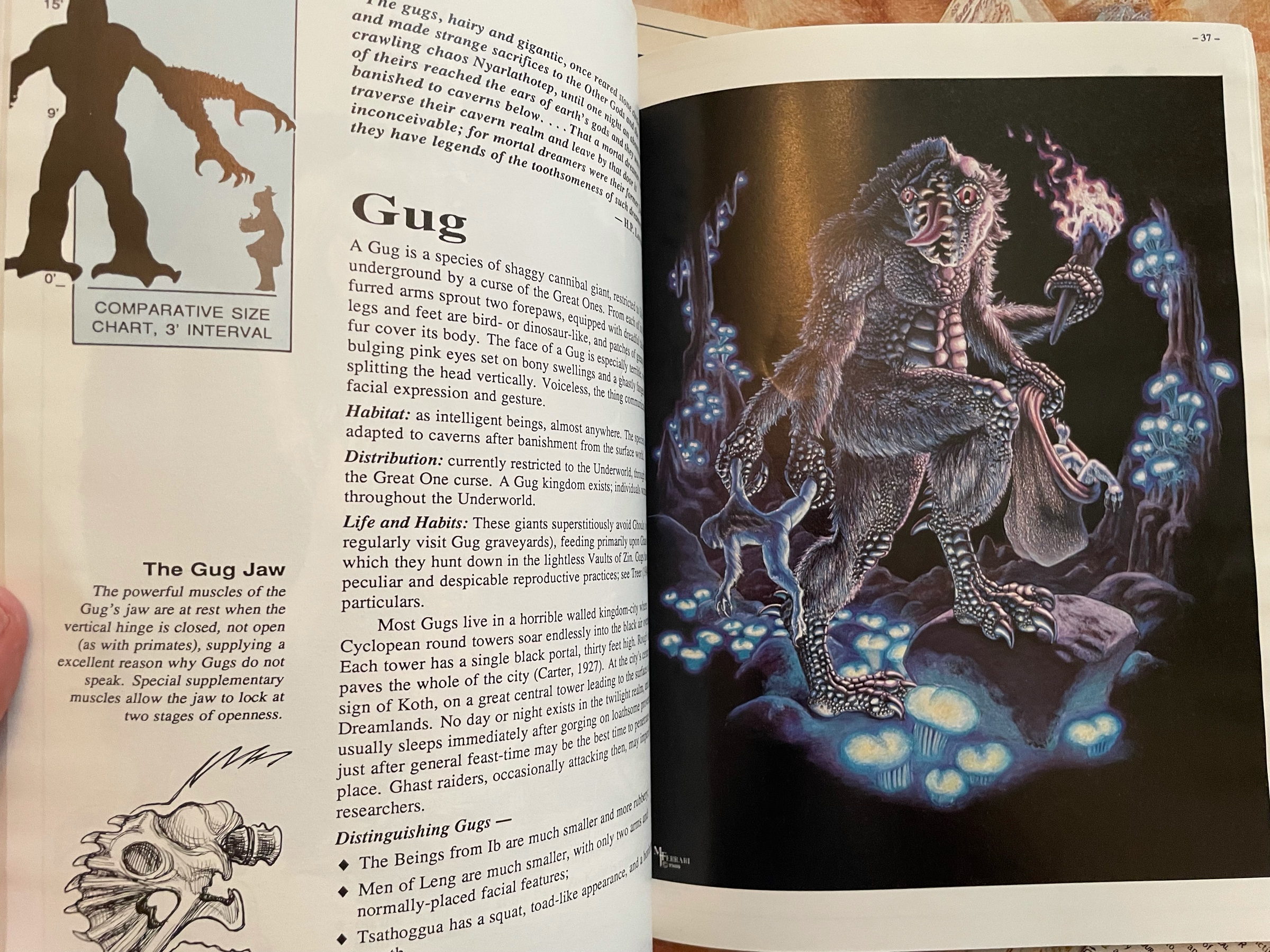
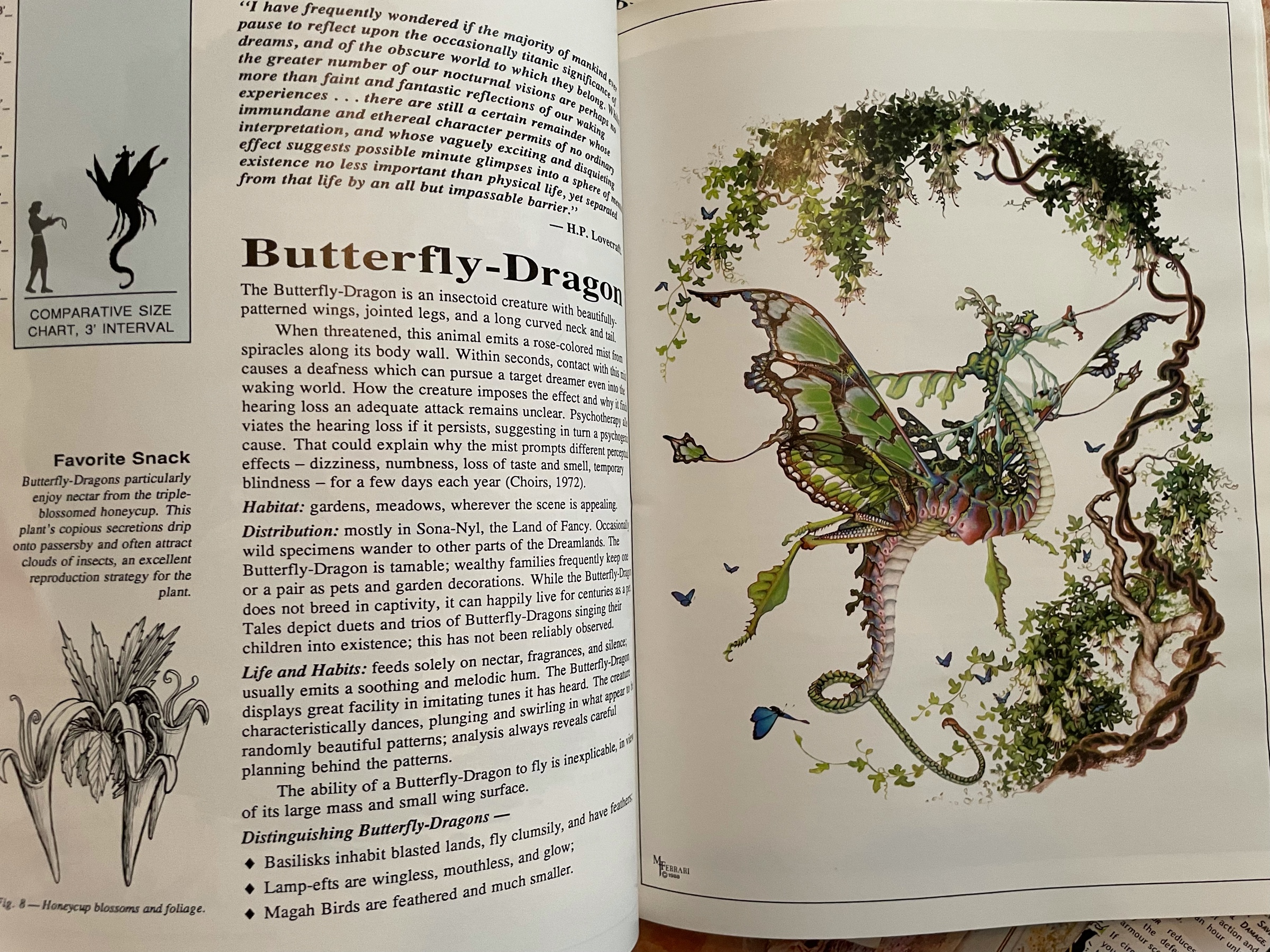
I already had the Dreamlands one in French, but I recently grabbed both in English (they had a decent price on eBay!) For a long time it was my only systemless RPG supplement and I loved how that, with its weird illustrations, set it apart!
Harn’s Kingdom of Kaldor in hardback! The printing quality is top notch and the paper smells good. I love this kind of detailed material, and the maps are always awesome.
We have just watched Back To The Future 2 with the oldest kid and it still rules! I absolutely love the trilogy unconditionally. The shot where Marty comes running back in 1955 as Doc just sent him away is sooooo goood
I don’t get to play hexcrawls very much at all but I love to open some page at random and get some cool idea for my ongoing game, whatever it is!
Weirdness double whammy! I have only skimmed those two but I like what I’m seeing…
The Dragon is now keeping company to the Seasons of Sartar! The cult initiations ideas are worth the price of the book alone in my opinion…
Got some vintage Call of Cthulhu books… some people don’t like Fatal Experiments, but Tatterdemalion was my first encounter with King In Yellow reality warping so it’s got some nostalgia factor!
Given the amount of preparation I’ve done for tonight’s Delta Green one-shot (scheduled to last two sessions), I think I’ll mostly do what Dennis Detwiller called “Seat of the Pants RPGing”… https://www.delta-green.com/
The Matrix Resurrections trailer looks good but it’s all stuff we’ve seen before. It feels like this movie will have a bad case of The Force Awakens, and possibly even worse because it doesn’t even introduce new protagonists and villains.
Old school GURPS! I love those character sheets.