From Archos to Amazon
As you probably already know, Amazon’s tablet, the Kindle Fire, was announced a few days ago. Priced at a pretty amazing $199, its purpose is more focused than your general usage tablet like the iPad or the Xoom: it’s specifically designed for consuming content like books, music and video (preferably through Amazon’s own services, of course). Although it will probably be possible to install some other apps (through Amazon’s AppStore) to do some email and chatting and gaming and what have you, it will probably be more limited than on those bigger tablets, and will likely be only advertised as the last bullet point on the list of specs, if at all.
Now you know what else was priced around $200 and was useful mostly for consuming content like books, music and video? Yep. The Archos 70 I got last year for $249 (I got it on a discount, it was normally at $279).
Oh sure, Archos may not have been advertising their product like that. They may have advertised it along the lines of:
The most awesome Android tablet ever! Do everything you want! Web browsing! Videos and music! Email!! Games!!! Video chat!!!! This is so awesome we’re running out of exclamation marks!!!!!
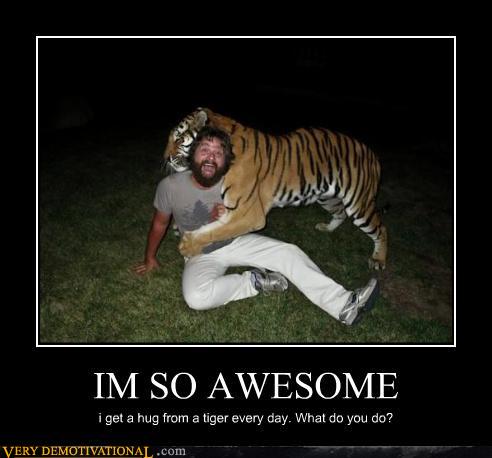
Being a long time Archos user, I’m used to their bullshit and I know how to read through it. It usually translates to:
It’s rubbish at everything, especially anything web-related, but it can read any fucking video format in the universe, doesn’t come with any bullshit iTunes-like sync program, and is so much cheaper than the competition.
And as I stated last time, that was just perfect: I only wanted a good portable media player, and maybe a nicer way to read my Instapaper clips. But, of course, everytime somebody saw my small cheap looking tablet, there was a good chance the discussion would go like this:
- “What’s that?”
- “My Archos 70. Android tablet. I use it mainly as a portable media player, though.”
- “Why didn’t you get an iPad?”
- “Too big. Not enough codec support. Too expensive.”
- “Yeah but you can do so much more with it!”
- “Don’t care.”
- “Come on! Apple is so awesome! The iPad is so shiny!”
- “Yeah, whatever.”
Well it looks like Amazon found a lot of people like me out there because the Kindle Fire’s product story is exactly what I was looking for a year ago. Well… apart for that one small detail about data freedom, because I’m not sure exactly how easy it will be to get your own content onto the device, without necessarily going through Amazon (especially since most of their content is not even available outside of the U.S. anyway).
At least I’m very happy to see someone try to move the market into a slightly new direction instead of spitting out confusing all-purpose Android tablets like everyone else.