Visual Studio Tips & Tricks
Stephen Walther recently blogged about tricks that every developer should know about Visual Studio. Most of those he mentioned I use on a daily basis, so I highly recommend them. It actually surprises me how many other programmers don’t know about those features. Somehow, most programmers know Visual Studio as much as they know Microsoft Word: there’s a big space in the middle to type your text, and then maybe they know a couple of menus and shortcuts and that’s it.
Anyway, here are a few other features I use frequently:
Tip #1 – Use CTRL-I for incremental search
I’m a big fan of incremental search. It’s actually the reason I originally switch from Internet Explorer to Firefox, back when Firefox was in beta (I already had tabs with Netcaptor). By the way, I’m happy to see IE8 now also features incremental search but, well, I’m hooked on FF now… anyway…
In Visual Studio, the incremental search mode is entered with CTRL-I. Then you can start typing right away what you want to find. You can also use backspace and all, as expected. The only problem is that it uses the last search mode defined in the “Find” dialog, so if the “Match case” option is currently checked, it will only perform incremental search on what you type in a case sensitive way. This may be a bit confusing at first, but using incremental search really makes navigating code faster.
Tip #2 – Use navigate backward and forward
Like the first tip, this tip make it faster to navigate code (because we’re spending more time reading code than writing code).
CTRL– (minus sign) and CTRL-SHIFT– allow you to respectively navigate backward and forward. The first one is the one I use almost all the time: I’m looking at some code, see a call to a function, go to the definition of that function, search for something, and then I want to go back to where I was at first. Well, without having to worry about anything, I can navigate backward twice and I end up where I want. No need to find the correct tab, figure out if I stayed in the same file or not while jumping through the code, etc. Wicked!
Tip #3 – Define some external tools
You can define “external tools” with, surprisingly, the Tools > External Tools menu item. A common thing for me is to define revision control related stuff there because most of the time I don’t like how buggy and slow source control plugins are. I also do this in Visual Studio Express where there is no support for plugins at all in the first place.
For example, say you’re using Perforce. You can create a new external tool like this:
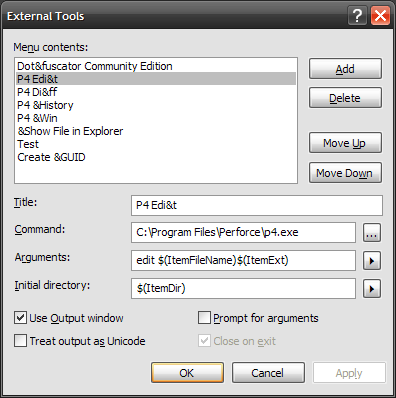
It will checkout the current file (the one you’re editing if the focus is in the text editor, or the one selected in the Solution Explorer if that’s where the focus is). Now you just need to bind “Tools.ExternalCommand2” (because it’s the second external tool) to the keyboard shortcut of you choice (mine is CTRL-K, CTRL-E for “open for edit”). Shazam! Just use your keyboard shortcut and you can checkout a file without leaving the text editor.
You can setup other commands, like show the history of the file or make a diff with the previous version. You can also easily adapt this to other systems, like SubVersion (except in this case you won’t need the “open for edit” command because SVN doesn’t lock files by default).